Understanding PDO in PHP: A Comprehensive Guide
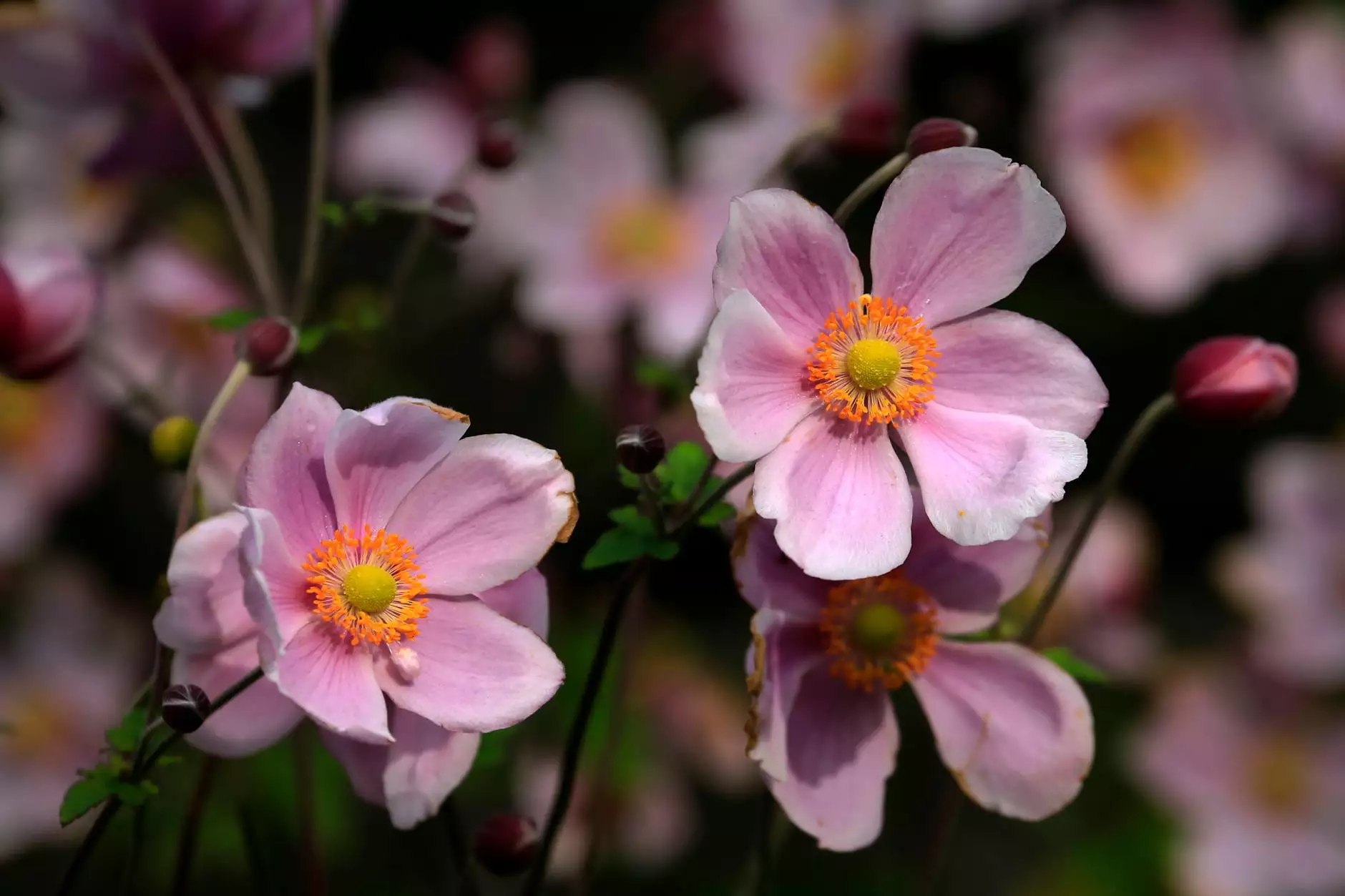
In the world of web development, programming languages like PHP play a crucial role, especially when it comes to database interactions. One of the most powerful tools available to PHP developers is PHP Data Objects (PDO). In this article, we will delve deep into what PDO is, how it works, and why it is essential for secure and efficient database operations. We will also explore the concept of parameterized strings, exemplified by the text pdo pdo :: param_str 0934225077, and how it ties into safe querying practice.
What is PDO?
PDO, or PHP Data Objects, is a database access layer that provides a uniform method to interact with various database systems. It abstracts the differences between databases, allowing developers to switch database management systems (DBMS) without changing the underlying codebase significantly. This feature is especially beneficial for applications that may need to support multiple databases over their lifecycle.
Core Features of PDO
- Database Agnosticism: PDO offers a consistent API for various databases including MySQL, PostgreSQL, SQLite, and more.
- Prepared Statements: Improves security and performance by allowing the same SQL statement to be executed multiple times with different parameters safely.
- Error Handling: PDO provides robust error handling methods that help developers easily troubleshoot and manage database interactions.
- Transaction Support: Complete support for transactions helps maintain data integrity during complex operations.
The Importance of Parameterized Queries
One of the standout features of PDO is its support for parameterized queries, which are essential for mitigating SQL injection risks. SQL injection is a critical security vulnerability that allows attackers to manipulate SQL queries by injecting malicious input.
What is a Parameterized Query?
A parameterized query is a type of SQL statement in which placeholders are used for parameters, making it safer and more efficient. When using parameterized queries, the database handles the escaping of special characters, which significantly reduces the risk of SQL injection. An example of a parameterized query might look something like this:
SELECT * FROM users WHERE phone_number = :phone_numberHow to Use PDO for Parameterized Queries
Implementing parameterized queries in PDO is straightforward. Here’s a step-by-step guide:
- Establish a Database Connection: Use the PDO constructor to connect to your database. $pdo = new PDO('mysql:host=localhost;dbname=testdb', 'username', 'password');
- Prepare the SQL Statement: Create a prepared statement with placeholders. $stmt = $pdo->prepare('SELECT * FROM users WHERE phone_number = :phone_number');
- Bind Parameters: Bind the parameters with actual values securely. $stmt->bindParam(':phone_number', $phone_number);
- Execute the Statement: Call the execute method to run the query. $stmt->execute();
Example: Using PDO with the Parameterized String 'pdo pdo :: param_str 0934225077'
Let’s take the example pdo pdo :: param_str 0934225077 to illustrate how to use a parameterized query effectively.
1. Database Connection
$pdo = new PDO('mysql:host=localhost;dbname=swansondental', 'username', 'password');2. Preparing the SQL Statement
$stmt = $pdo->prepare('SELECT * FROM users WHERE phone_number = :phone_number');3. Binding the Parameter
Here’s where we bind the phone number from our example:
$phone_number = '0934225077';$stmt->bindParam(':phone_number', $phone_number);4. Executing the Query
$stmt->execute();Once executed, you can fetch results safely without the threat of SQL injection.
Best Practices for PDO User Interactions
To fully leverage the capabilities of PDO, adhere to the following best practices:
- Always Use Prepared Statements: Even for simple queries, prepared statements add an additional layer of security.
- Leverage Error Modes: Set error modes to exceptions to catch issues early in the development process. $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- Close Connections: Although PHP closes connections automatically on script termination, explicitly closing connections can free up resources earlier.
Extending PDO Functionality
While PDO offers extensive built-in functionality, developers can extend its capabilities to fit their application’s unique needs. This can include creating custom classes that inherit from PDO or implementing additional methods that wrap common database interactions.
Creating a Custom PDO Class
A simple example of a custom PDO class might look something like this:
class Database extends PDO { public function __construct($dsn, $username, $password) { parent::__construct($dsn, $username, $password); $this->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } public function fetchUser($phone_number) { $stmt = $this->prepare('SELECT * FROM users WHERE phone_number = :phone_number'); $stmt->bindParam(':phone_number', $phone_number); $stmt->execute(); return $stmt->fetch(PDO::FETCH_ASSOC); } }Using a custom class not only organizes your code but also allows for easily reusable methods across your application.
The Future of PDO and Database Interactions
As technology evolves, so does the landscape of web development. PDO remains a robust choice for PHP developers, combining simplicity with powerful features for effective database management. With growing data privacy regulations and security concerns, the importance of secure database interactions cannot be overstated.
Keeping Up with PDO Updates
To stay ahead in the industry, developers must keep abreast of updates and enhancements to PDO, particularly as new threats emerge and database technologies evolve. Following best practices and engaging with community resources can help maintain a secure and efficient development environment.
Conclusion
In summary, understanding and effectively utilizing PDO is essential for any PHP developer focused on delivering secure and high-performance web applications. By embracing features like parameterized queries, as shown with the example of pdo pdo :: param_str 0934225077, developers can enhance the robustness of their applications against common vulnerabilities. At Swanson Dental, having robust data management capabilities can significantly contribute to maintaining patient records, processing appointments, and ensuring overall operational efficiency in the dental field.
Remember, adopting best practices like those discussed in this article will not only safeguard your applications but also streamline interaction with your databases, making your development process more effective and sustainable.